In this module we will talk about some of advance features that spring data JPA support for developing an enterprise application. They are paging and sorting ,auditing, locking and custom repository.
Paging and Sorting
As a web application developer if you have to display thousands of records on the UI it is not a good practice to just query database and display it to UI. Spring data JPA provides paging and sorting mechanism to handle such type of scenario. These are most sought problem if you are dealing with a big enterprise application, keeping this into mind spring data JPA has provided in built support for paging and sorting.
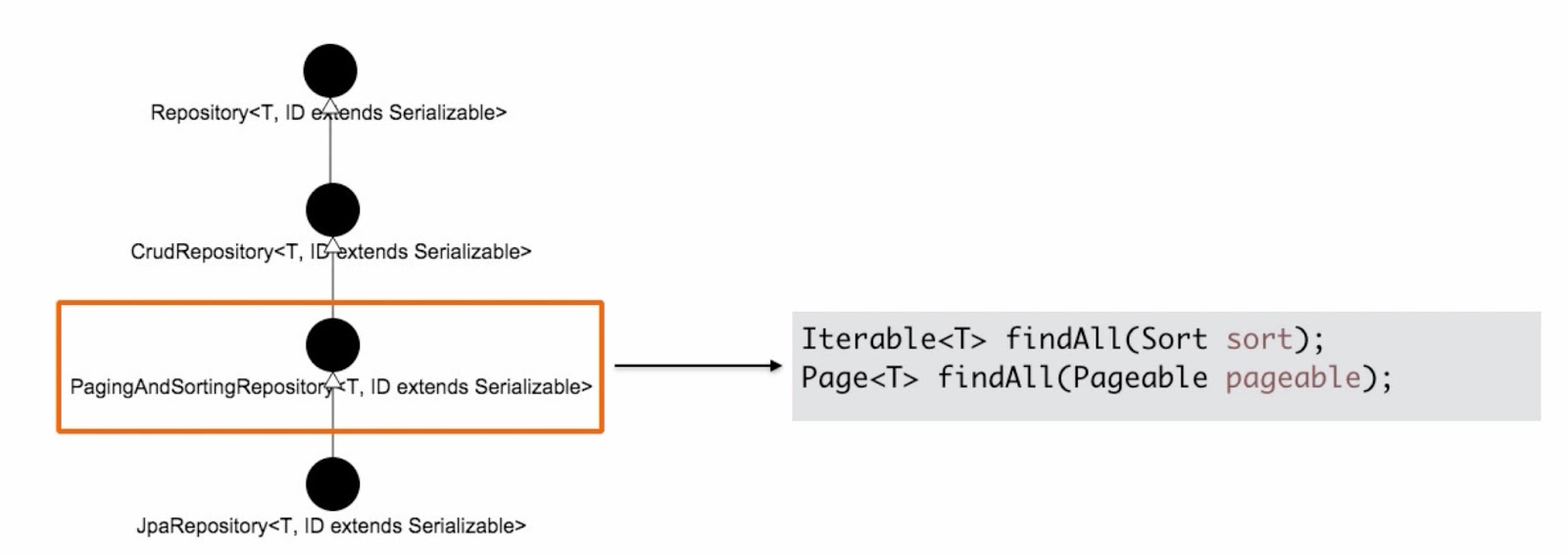
You can see above that spring data JPA has PagingAndSortingRepository interface and it has findAll method. Sort and Pageable object are getting passed as part of findAll() method parameters. What do you think Pageable does behind the scene. Basically Pageable is an interface and when spring data JPA fetch value from database it limits the result. So internally spring data JPA adds 'limit' keyword as part of query. And the limit would be define the parameters that we pass to Pageable object.
Likewise, Sort is an interface for sorting out the database query result. In this case internally spring data JPA adds 'order by' clause to result out in sorted order.
Let's see how do we implement paging and sorting with an example ,I am taking PromotionTypeJpaRepository interface -

You can see here that method is returning Page object instead of List and Pageable object is being passed as method parameters. If we want create the Pageable object manually, the service class (or other component) that wants to paginate the query results, which are returned by a Spring Data JPA repository, must create the Pageable object and pass it forward to the invoked repository method.
PageRequest is the implementation for Page interface and it takes many parameterized constructor. In this case we are passing two integer value denoting first page with page size 2. If you think on it a bit then internally while forming query spring data JPA will add limit with 2 which will help to fetch only 2 rows from database.
Now if we have to sort the query results in ascending order by using the values of the
name field. If we want to get the second page by using page size 10, we have to create the Pageable object by using the following code:
Here we are describing the sort direction by creating Sort object and passing Direction into it. If we want to sort the query results in ascending order, we have to append the keyword Asc to the method name of our query method. On the other hand, if we want to sort the query results in descending order, we have to append the keyword Desc to the method name of our query method.
Internally spring data JPA will add
order by clause while query in database to implement sorting.
Custom Repository
One of the very important concept that I am going to discuss here is to create a custom repository. In an enterprise application it is most viable to add custom method apart from the one that spring data JPA provides.
It is shown in the above picture the best practice to create a custom repository. I will explain this by using an example-
First create a MyJpaRepositoryCustom interface with a customMethod() as follow :
Create MyJpaRepository which implements JpaRepository and have MyJpaRepositoryCustom interface implemented into it-
Now while creating the implementation for custom interface you must have name ending with 'Impl' with custom JpaRespository in this case name would be 'MyJpaRepositoryImpl' -
The above class will have implementation for all methods defined in custom interface and also have access for JpaRepository methods. At application starts up spring data JPA would look for implementation of MyJpaRepository interface with 'Impl' added into it(MyJpaRepositoryImpl). So in any of spring component or in Service class if custom interface methods are called it automatically call the implementation defined in 'Impl' class.
Auditing Support
When you are dealing with large scale enterprise data then it is very likely to know that "when the data was created ?" ,"who created the data ?" ,"when was it last modified ?". Spring data JPA also provide support for auditing by using annotation. Following annotations are used by spring data JPA for auditing-
- @CreatedBy : It can be used if there is an User type data.
- @CreatedDate : It is used for DateTime data type.
- @LastModifiedBy : It is used to give information about last modified data with User type.
- @LastModifiedDate : It is used for last modified DateTime data type.
All these annotations are used on the instance variables of an entity. Spring also provides an AuditorAware interface for auditing purpose.
You can see that Java class for configuration is being used and AuditorAware bean has been set up. To perform auditing you have to enable spring data JPA auditing by using @EnableJpaAuditing.
Locking
Locking is very critical concept when you are dealing with data access layer. Locking is set up for a table when multiple user trying to modify the concurrently access data. Spring data JPA provides @Version annotation to implement locking strategy as below .
Whenever any data is being modified by multiple user at the same time ,the persistence manager checks for the variable annotated with @Version inside an entity. Every time data is modified persistence manager update the value of variable annotated with @Version. When you set up locking the persistence manager will check the latest version of an entity and prevent the entity to modify.
There are two type of locking -
- Optimistic locking : If version number doesn't match throw OptimisticLockException.
- Pessimistic locking : When this type of locking is used it locks the data for long term for the transaction duration, preventing others from accessing the data until the transaction commits.
The locking can be implemented by using @Lock annotation as below :
We provide @Lock annotation on query methods in custom Jpa Repository and pass LockModeTye parameters for the type of locking.
As spring data JPA is a very vast topic hence I have covered it by multiple post. In order to learn and understand spring data JPA completely you need go through each post. Below mentioned order you must follow so that you won't miss anything-
Comments
Post a Comment